Currying is transformation of a function that translate a function from callable as f(a,b,c) into callable as f(a)(b)(c). Currying doesn’t call a function it just transform it.
In other words we can say that Currying is breaking down function with multiple arguments one into one or more functions that each accept a single argument.
/**
syntax for Currying
*/
f(a,b,c) => f(a)(b)(c)
Simple Example for Multiplying Numbers
/**
Simple example for multiplying numbers
*/
const multiplyNums = (a, b, c) => a * b * c;
console.log("MultiplyNums ::", multiplyNums(5, 6, 7));
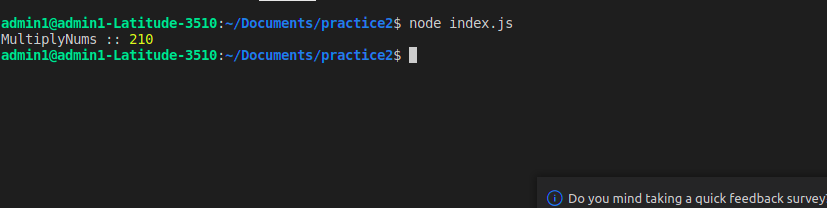
After Applying Currying
/**
Simple example for multiplying numbers after using Currying
*/
const curriedMultiplyNums = (a) => (b) => (c) => a * b * c;
console.log("Currying Multiply Nums ::",curriedMultiplyNums(6)(7)(8))
